Queries
Loading "Queries (π solution)"
Run locally for transcripts
I'm going to start by locating the discount code input on the page. This one:
<label htmlFor="discountCode" className="mb-1 block">
Discount code
</label>
<input
id="discountCode"
name="discountCode"
className="w-full rounded-md border px-2 py-1 focus:ring-4"
placeholder="ABCD1234"
pattern="[A-Z]{4}[0-9]{4}"
autoComplete="off"
required
/>
A user would find this input by its label text because they would see that it says "Discount code" above that input. When creating the
<DiscountCode />
component, I've made sure to have accessible markup by associating a label
element with the input by its id
:<label
htmlFor="discountCode"
className="mb-1 block"
>
Discount code
</label>
<input
id="discountCode"
name="discountCode"
className="w-full rounded-md border px-2 py-1 focus:ring-4"
placeholder="ABCD1234"
pattern="[A-Z]{4}[0-9]{4}"
autoComplete="off"
required
/>
I can then select this input by using the
.getByLabelText()
method on the page
object from @vitest/browser/context
:import { page } from '@vitest/browser/context'
const discountInput = page.getByLabelText('Discount code')
π¦ The.getByLabelText()
query looks up an element by the label text associated with that element. The label text can be the text of an explicit<label>
associated with the element or the element'saria-label
attribute value.
Now to make sure that the input is visible for the user, I will write an assertion using
expect.element()
and the .toBeVisible()
matcher:await expect.element(discountInput).toBeVisible()
π¦ You can also chain locators to access more specific elements. For example,page.getByRole('navigation').getByRole('link', { name: 'My projects' })
is a great way to find a "My projects" link that is nested under therole="navigation"
/<nav>
parent, especially if there are multiple links with the same accessible name on the page.
So far so good!
Next on the list is the button that makes the whole form do somethingβthe submit button. While I can select it by its text
'Apply discount'
, that would be quite a broad query. There can be multiple elements with that same text on the page (e.g. a heading that also says "Apply discount"). A far more important desctiptor for this element is that it is a button.With that in mind, I will locate the button by its role and then its accessible name:
const applyDiscountButton = page.getByRole('button', {
name: 'Apply discount',
})
π¦ The.getByRole()
query looks up an element (or elements) by its role. Additionally, you can narrow down the selector to include the element's accessiblename
(don't confuse with the element's text!).
And in the similar fashion, I will add an assertion for the submit button's presence on the page:
await expect.element(applyDiscountButton).toBeVisible()
This concludes this test case, but there's more when it comes to the best practices of writing queries. Let's take a close look at those.
Choosing queries
When testing your components, you may find yourself in a position where multiple queries can be used to target an element. Or, in an opposite twist of events, you may be unsure which query to use at all.
In either case, there's a rule of thumb that you can follow:
Query specificity is similar to specificity of CSS selectors. For example, the
div
selector is not very specific as there can be multiple <div>
elements on the page, while the #header
selector is highly specific, pointing to a single element with that ID.Element queries in your component tests are much the same. You can grade them by specificity:
getByTestId()
is generally discouraged. It is not accessible and doesn't resemble how your users perceive the page. You are better off with a more specific query 99% of the time.Accessible name vs text content
The
getByRole()
query allows you to specify the accessible name
of an element. It's important to stress that accessible name and element's text content are not the same thing.Accessible name
Accessible name is the name of an element that describes its purpose or intent. A lot of HTML elements get their accessible name from their text content:
<a href="/">Homepage</a>
page.getByRole('link', { name: 'Homepage' })
<button>Add to cart</button>
page.getByRole('button', { name: 'Add to cart' })
But there are also elements that don't. Some derive their accessible name from text content of associated elements (like
<input>
gets it name from the associated <label>
text) while others get it from its attributes (e.g. the name for an <img>
is taken from its alt
attribute).π Learn more about where different HTML elements get their accessible names in the Accessible name calculation.
And then there are elements that don't need accessible name at all. Those include
<section>
, <p>
, <span>
, or images that are purelly illustrational.Text content
Text, or child content is the inner text of an element:
<p>Welcome back!</p>
page.getByRole('???', { name: 'Welcome back!' }) // β
page.getByText('Welcome back!) // β
General text elements do not have a role or an accessible name, so selecting them by text is your best option.
By-text queries are tremendously useful to locate elements the user (or a screen reader) would locate by their text content.
That being said, note that some text elements can have specialized roles, and in that case you should prefer
getByRole()
:<p role="alert">Subscription canceled</p>
π¦ While this paragraph has an explicitalert
role to announce important change to the user, it still doesn't have an accessible name.
Looking up roles and names
This all may seem a bit overwhelming. Don't worry, you are not required to learn the whole ARIA specification by heart. Start small and improve as you go.
To help you on that journey, remember that you can observe the list of roles and accessible names for the markup your components are rendering right now. This can help tremendously, especially when you cannot figure out why your test fails to locate a seemingly present element.
You can list the accessible roles and names in two ways: directly in your test, and in your browser's DevTools.
In your tests, take advantage of the
logRoles()
function from the @testing-library/dom
package:import { logRoles } from '@testing-library/dom'
test('renders the navigation', () => {
render(
<nav>
<ul>
<li>
<a href="/">Home</a>
<a href="/blog">Blog</a>
</li>
</ul>
</nav>,
)
logRoles()
})
navigation:
Name "":
<nav />
--------------------------------------------------
list:
Name "":
<ul />
--------------------------------------------------
listitem:
Name "":
<li />
--------------------------------------------------
link:
Name "Home":
<a
href="/"
/>
Name "Blog":
<a
href="/blog"
/>
--------------------------------------------------
This prints you a list of element roles and their accessible names to use in your test.
Some browsers provide you the devtools to help you see the accessible tree of your application. For example, in Chrome you can go to "Elements -> Accessibility" (in the dropdown menu next to "Styles" and "Computed") to see all the available roles and names rendered in your application:
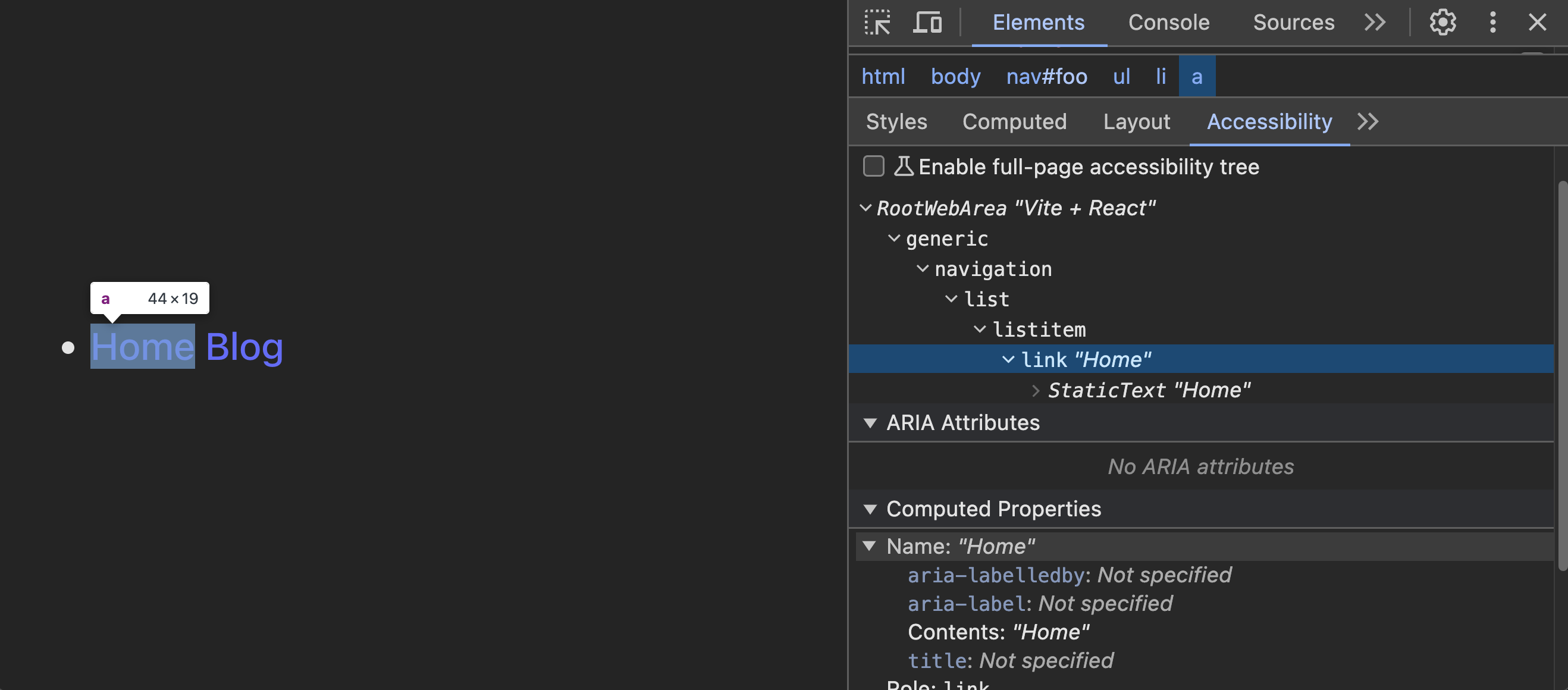
π¦ Learn more about the Accessibility features in Chrome.
Role of accessibility in testing
The grand question here might be: Why go through all of this just to write some tests?
But here's the thing. Nothing you've just learned is about testing. It's about accessibility. Tools like Vitest Browser Mode or React Testing Library simply leverage accessible markup, encouraging you to write one.
At worst, you are getting a test that is decoupled from its implementation details. Accessibility roles, names, and attributes are not implementation details because you aren't writing them for yourself. You are writing them for your users. Your component must have its accessible elements regardless of how it's implemented, and so should your tests.
At best, you are getting implicit accessibility testing for free. Forgot to set the
for
attribute on the <label>
? Oops, the test is failing because nobody can access that associated input anymore!